SFTP is a simple and fairly reliable way to share the information within the organization. Let’s look at the situation when you need to pick up some files from a remote host with authorization by public key. And after that, let’s see how to use it with in python.
Moreover, let’s see how to work with SSH using python and execute any commands on the remote host. For example. if we need it to collect versions of installed packages and a version Linux distribution for further vulnerability analysis (see “Vulnerability Assessment without Vulnerability Scanner“). 😉
Generating public key:
cd ~ mkdir .ssh chmod 700 .ssh $ ssh-keygen Generating public/private rsa key pair. Enter file in which to save the key (/home/vmuser/.ssh/id_rsa): [PRESS ENTER] Enter passphrase (empty for no passphrase): [INPUT p@$phr4se PRESS ENTER] Enter same passphrase again: [INPUT p@$phr4se PRESS ENTER] Your identification has been saved in /home/vmuser/.ssh/id_rsa. Your public key has been saved in /home/vmuser/.ssh/id_rsa.pub. The key fingerprint is: 52:14:a4:33:71:0a:b9:46:25:73:a0:96:94:b3:3b:03 vmuser@localhost.localdomain The key's randomart image is: +--[ RSA 2048]----+ | ..=++.=. | | .ooo= * | | ++ .= . | |E.. o + | | . o . S | | + . | | o | | | | | +-----------------+
Here is the public key id_rsa.pub, which we send to the server owner, who will add it to the list of known keys:
$ ls /home/vmuser/.ssh/ id_rsa id_rsa.pub known_hosts
Once he does this, we can go to that host by SSH:
[vmuser@localhost pycharm-community-2017.1.3]$ ssh 192.168.56.20 Enter passphrase for key '/home/vmuser/.ssh/id_rsa': Last login: Mon Sep 3 10:50:10 2017 from 192.168.56.101 ...
And how download the files on the host from the python script? You will need to install pysftp:
# sudo pip install pysftp
To connect and download files from the ‘data/’ directory on the remote server to the local directory ‘data/’ we need to do something like this:
import pysftp sftp = pysftp.Connection(host = '192.168.56.20', private_key = '/home/vmuser/.ssh/id_rsa', private_key_pass = 'p@$phr4se') for file in sftp.listdir("data"): sftp.get(remotepath = "data/" + file, localpath="data/" + file )
Ok, we dealt with the downloading. Now let’s see how to execute commands with SSH in python using paramiko module. When we installed pysftp we also installed paramiko by dependencies. We can use authentication by keys and by password (commented):
#!/usr/bin/env python import paramiko command = 'cat /etc/centos-release; rpm -qa' try: client = paramiko.SSHClient() client.load_system_host_keys() client.set_missing_host_key_policy(paramiko.WarningPolicy) client.connect(hostname = '192.168.56.20', key_filename='/home/vmuser/.ssh/id_rsa', password = 'p@$phr4se') #client.connect(hostname = '192.168.56.20', username="vmuser", password="1") stdin, stdout, stderr = client.exec_command(command) And having a list of packages we can check them for vulnerabilities using your own scanner or Vulners API. print(stdout.read()) print(stderr.read()) finally: client.close()
The output will be like this:
CentOS Linux release 7.3.1611 (Core) NetworkManager-team-1.4.0-12.el7.x86_64 centos-release-7-3.1611.el7.centos.x86_64 NetworkManager-wifi-1.4.0-12.el7.x86_64 filesystem-3.2-21.el7.x86_64 lvm2-2.02.166-1.el7.x86_64 ncurses-base-5.9-13.20130511.el7.noarch kexec-tools-2.0.7-50.el7.x86_64 bind-license-9.9.4-37.el7.noarch rdma-7.3_4.7_rc2-5.el7.noarch ...
And having a list of packages we can check them for vulnerabilities using your own scripts or Vulners Audit API.
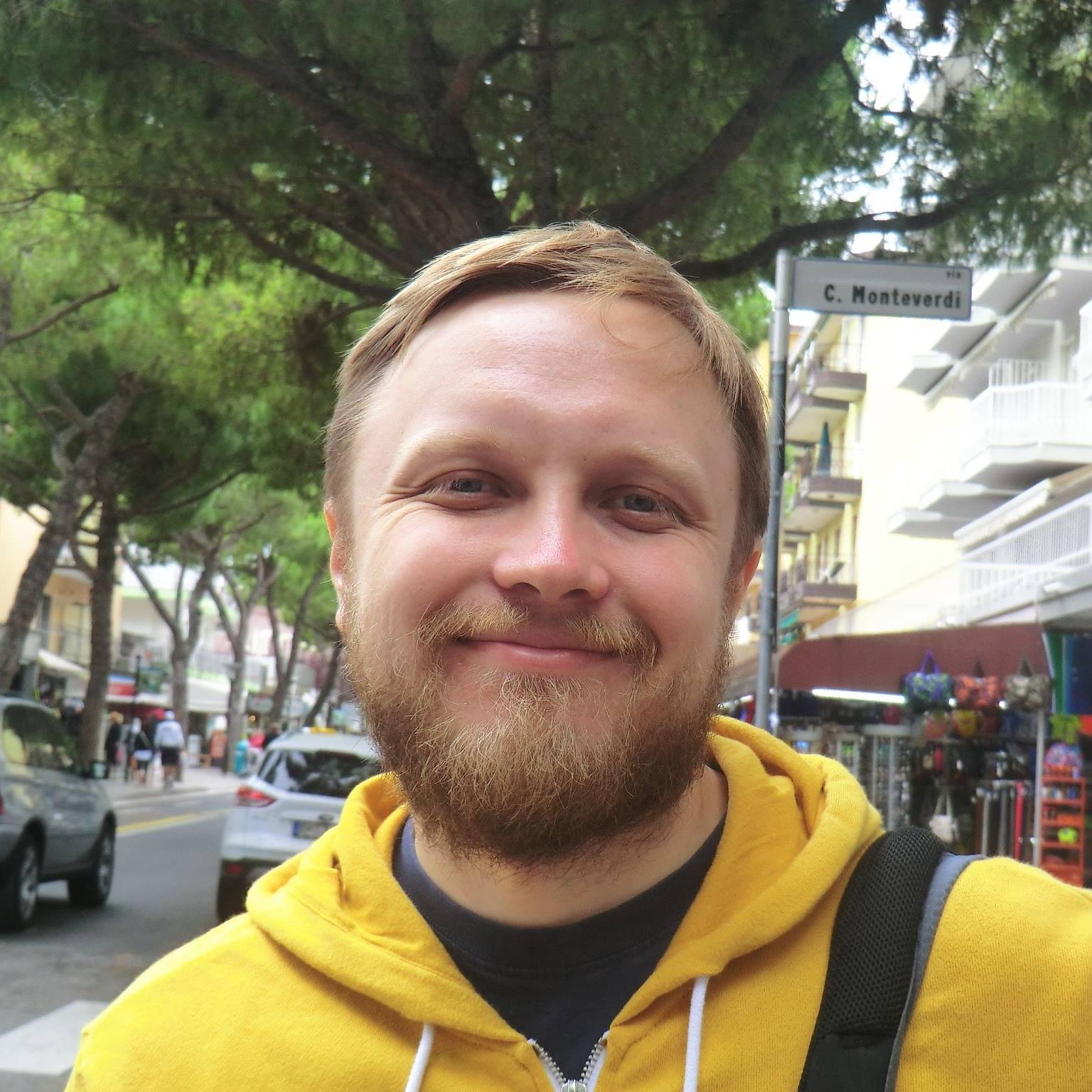
Hi! My name is Alexander and I am a Vulnerability Management specialist. You can read more about me here. Currently, the best way to follow me is my Telegram channel @avleonovcom. I update it more often than this site. If you haven’t used Telegram yet, give it a try. It’s great. You can discuss my posts or ask questions at @avleonovchat.
А всех русскоязычных я приглашаю в ещё один телеграмм канал @avleonovrus, первым делом теперь пишу туда.
…or you can use Ansible for that 😉
Pingback: Making Expect scripts for SSH Authentication and Privilege Elevation | Alexander V. Leonov