Atlassian Jira, Python and automated labeling. I have already wrote about Atlassian Jira automation in “Automated task processing with JIRA API“. But all examples there were with using of curl. So, I decided to make one more post about Jira API. This time with python examples and about labeling issues (nice wordplay, right? ).
You can use labeles for organizing issues on Jira Scrum and Kanban Boards, Jira Dashboards or just for advanced searching (e.g. labels = "LabelName"
)
Let’s start from the basics.
How to search Jira issues from your own python scripts?
It’s easy. Send a post request to /rest/api/2/search/ with some JQL expression. Jira server will return first 50 matching issues. If you need more, set a startAt parameter and repeat post requests while the number of issues you requested is less than total number of founded issues (parameter in response).
Something like this:
import requests
import json
requested = 0
search_results = dict()
total = 1
while requested < total:
post_data = json.dumps({'jql': 'assignee in (user_id) order by created DESC', "startAt": requested, "maxResults": 50})
headers = {
'Content-Type': 'application/json',
}
response = requests.post('https://jira.corporation.com/rest/api/2/search/', headers=headers, data=post_data,
verify=False, auth=(user, password))
data = json.loads(response.text)
total = data['total']
search_results[requested] = data
requested += 50
Get the issue IDs from the search_results structure:
for base in search_results:
for key in search_results[base]['issues']:
print(key['key'])
Output:
SECURITY-317
SECURITY-314
SECURITY-313
SECURITY-498
SECURITY-497
SECURITY-402
SECURITY-401
SECURITY-400
SECURITY-489
SECURITY-482
How to get issue date knowing it’s id?
You can do it by sending a get request to /rest/api/2/issue/[issue id]:
issue_id = "SECURITY-498"
headers = {
'Content-Type': 'application/json',
}
response = requests.get('https://jira.corporation.com/rest/api/2/issue/' + str(issue_id), headers=headers,
verify=False, auth=(user, password))
data = json.loads(response.text)
Here are the labels:
print(data['fields']['labels'])
Output:
[u'ImportantLabel']
How to edit the labels of Jira issue?
Create a small json with a part of issue data that you want to change and make a post request to /rest/api/2/issue/[issue id]
headers = {
'Content-Type': 'application/json',
}
new_labels = [ 'ImportantLabel', 'TestLabel' ]
put_data = json.dumps({ 'fields': { 'labels' : list(new_labels) } })
response = requests.put('https://jira.corporation.com/rest/api/2/issue/' + str(issue_id), headers=headers, data=put_data,
verify=False, auth=(user, password))
You can set other Jira fields in a similar way.
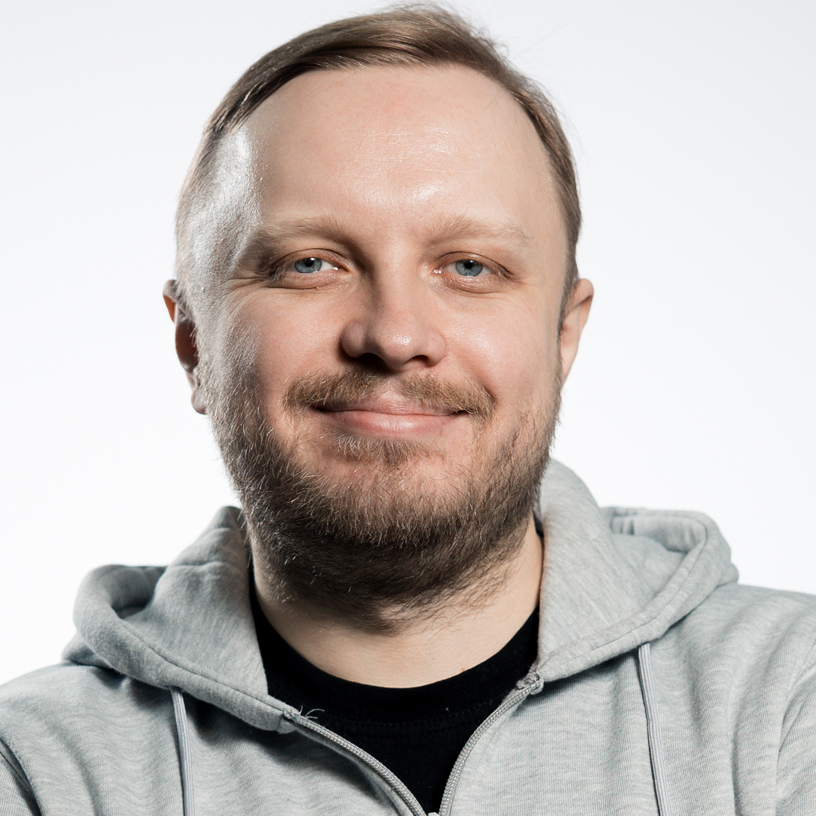
Hi! My name is Alexander and I am a Vulnerability Management specialist. You can read more about me here. Currently, the best way to follow me is my Telegram channel @avleonovcom. I update it more often than this site. If you haven’t used Telegram yet, give it a try. It’s great. You can discuss my posts or ask questions at @avleonovchat.
А всех русскоязычных я приглашаю в ещё один телеграмм канал @avleonovrus, первым делом теперь пишу туда.
Pingback: Confluence REST API for reading and updating wiki pages | Alexander V. Leonov
I tried exactly the last method to update labels, but I got server response 400.
I tried updated component field and it works well, here is my code for component field update
put_data = ‘{“update” : {“components” : [{“add” : {“name” : “%s”}}]}}’ % component
any help on this?